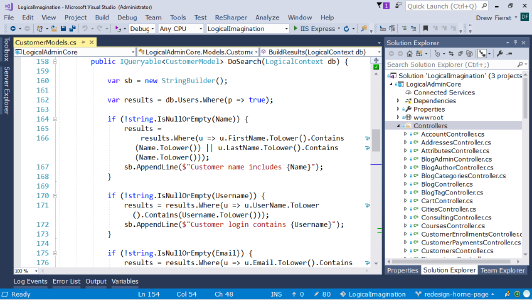
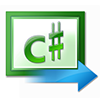
C# Essentials Training
2 days (10:00 AM - 5:00 PM Eastern)
$750.00
Register for a live online class.
Details
Subjects Covered
Prerequisites
Setup Requirements
Details
Course Details
Microsoft .NET is an advance in programming technology that greatly simplifies application development, both for traditional, proprietary applications and for the emerging paradigm of Web-based services. .NET 6 is a unified platform, for browser, cloud, desktop, IoT, and mobile apps. It is based on .NET Core, the package-based implementation that is cross-platform, running on Mac and Linux besides Windows. It completes the unification of the .NET platform begun with .NET 5.
This two-day course is designed for the experienced programmer to help you quickly come up to speed on the C# language. It is current to Visual Studio 2022, .NET 6, and C# 10. Important newer features such as dynamic data types, named and optional arguments, tuples, asynchronous programming keywords, nullable reference types, record types, and top-level statements are covered.
This course concisely covers the essentials of programming using Microsoft’s C# programming language. It starts with an overview of .NET architecture and the basics of running C# programs in a .NET environment. The following two chapters cover C# language essentials and object-oriented programming in C#. The next chapter discusses how C# relates to .NET. The following chapter covers delegates and events. The course includes a succinct introduction to creating GUI programs using Windows Forms. The course concludes with a chapter covering the newer features of C#. Appendices provide a tutorial on Visual Studio 2022, an overview of LINQ, and coverage of unsafe code and pointers in C#.
The course is practical, with many example programs and a progressively developed case study. The goal is to quickly bring you up to speed in writing C# programs. The student will receive a comprehensive set of materials, including course notes and all the programming examples.
Subjects Covered
C# Essentials
- Introduction to .NET
- What is .NET?
- .NET Framework, .NET Core, and .NET 6
- Application Models
- Managed Code
- Visual Studio 2022
- Console Programs and New Console Template
- GUI Programs
- C# Overview for the Sophisticated Programmer
- First C# Console Application
- Namespaces
- Data Types
- Conversions
- Control Structures
- Subroutines and Functions
- Parameter Passing
- Strings
- Arrays
- Implicitly Typed Variables
- Console I/O
- Formatting
- Exception Handling
- Object-Oriented Programming in C#
- Classes
- Access Control
- Methods and Properties
- Asymmetric Accessor Accessibility
- Static Data and Methods
- Constant and Readonly Fields
- Auto-Implemented Properties
- Inheritance
- Overriding Methods
- Abstract Classes
- Sealed Classes
- Access Control and Assemblies
- C# and the .NET Framework
- Components
- Interfaces
- System.Object
- .NET and COM
- Collections
- IEnumerable and IEnumerator
- Copy Semantics in C#
- Generic Types
- Type-Safe Collections
- Object Initializers
- Collection Initializers
- Anonymous Types
- Attributes
- Delegates and Events
- Delegates
- Anonymous Methods
- Lambda Expressions
- Random Number Generation
- Events
- Introduction to Windows Forms
- Creating Windows Applications Using Visual Studio 2022
- Partial Classes
- Buttons, Labels, and Textboxes
- Handling Events
- Listbox Controls
- Newer Features in C#
- Dynamic Data Type
- Named and Optional Arguments
- Variance in Generic Interfaces
- Asynchronous Programming Keywords
- New Features in C# 6 and C# 7
- Nullable Reference Types
- Record Types
- Top-Level Statements
- Using Visual Studio 2022
- Signing in to Visual Studio
- Overview of Visual Studio 2022
- Creating a Console Application
- Project Configurations
- Debugging
- Multiple-Project Solutions
- LINQ
- What Is LINQ?
- Basic Query Operators
- Filtering
- Ordering
- Aggregation
Prerequisites
Before Taking this Class
The student should be an experienced application developer or architect. Some background in object-oriented programming would be helpful.Setup Requirements
Software/Setup For this Class
Microsoft Visual Studio 2022 and Windows 10 or higher. The free Visual Studio Community 2022 can be used.
Onsite Training
Do you have five (5) or more people needing this class and want us to deliver it at your location?