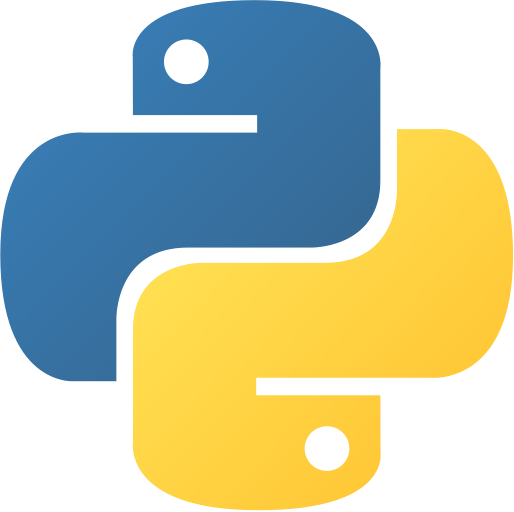
Python Introduction Training
4 days (10:00 AM - 5:00 PM Eastern)
$1,725.00
Register for a live online class.
Details
Subjects Covered
Prerequisites
Setup Requirements
Details
Course Details
This course leads you from the basics of writing and running Python scripts to more advanced features such as file operations, regular expressions, working with binary data, and using the extensive functionality of Python modules. Extra emphasis is placed on features unique to Python, such as tuples, array slices, and output formatting.
This is a hands-on programming class. All concepts are reinforced by informal practice during the lecture followed by graduated lab exercises. Python Programming is a practical introduction to a working programming language, not an academic overview of syntax and grammar. Students will immediately be able to use Python to complete tasks in the real world.
Subjects Covered
Python Introduction
- An Overview of Python
- What is Python?
- The Birth of Python
- About Interpreted Languages
- Advantages of Python
- Disadvantages of Python
- How to get Python
- Which Version of Python?
- The End of Python 2
- Getting Help
- The Python Environment
- Starting Python
- If the Interpreter is not in your PATH
- Using the Interpreter
- Trying Out a Few Commands
- Running Python Scripts
- Using pydoc
- Python Editors and IDEs
- Getting Started
- Using Variables
- Keywords and Built-ins
- Variable Typing
- Strings
- Single-delimited String Literals
- Triple-delimited String Literals
- Raw String Literals
- Unicode Characters
- String Operators and Methods
- Numeric Literals
- Math Operators and Expressions
- Converting Among Types
- Writing to the Screen
- String Formatting
- Legacy String Formatting
- Command Line Parameters
- Reading from the Keyboard
- Flow Control
- About Flow Control
- What’s with the white space?
- if and elif
- Conditional Expressions
- Relational Operators
- Boolean Operators
- while loops
- Alternate ways to exit a loop
- Array Types
- About Array Types
- Lists
- Indexing and Slicing
- Iterating through a Sequence
- Tuples
- Iterable Unpacking
- Nested Sequences
- Functions for all Sequences
- Using enumerate()
- Operators and Keywords for Sequences
- The Range() Function
- List Comprehensions
- Generator Expressions
- Working with Files
- Text file I/O
- Opening a Text File
- The with block
- Reading a Text File
- Writing to a Text File
- Dictionaries and Sets
- About Dictionaries
- When to Use Dictionaries
- Creating Dictionaries
- Getting Dictionary Values
- Iterating Through a Dictionary
- Reading File Data into a Dictionary
- Counting with a Dictionary
- About Sets
- Creating Sets
- Working with Sets
- Functions
- Defining a Function
- Returning Values
- Function Parameters
- Variable Scope
- Sorting
- Sorting Overview
- The sorted() Function
- Custom Sort Keys
- Lambda Functions
- Sorting Nested Data
- Sorting Dictionaries
- Sorting in Reverse
- Sorting Lists in Place
- Errors and Exception Handling
- Syntax Errors
- Exceptions
- Handling Exceptions with try
- Handling Multiple Exceptions
- Handling Generic Exceptions
- Ignoring Exceptions
- Using else
- Cleaning up with finally
- Using Modules
- What is a Module?
- The import Statement
- Where did __pycache__ come from?
- Module Search Path
- Packages
- Module Aliases
- When the Batteries aren’t Included
- Regular Expressions
- Regular Expressions
- RE Syntax Overview
- Finding Matches
- RE Objects
- Compilation Flags
- Groups
- Special Groups
- Replacing Text
- Replacing with a Callback
- Splitting a String
- Using the Standard Library
- The sys Module
- Interpreter Information
- STDIO
- Launching External Programs
- Paths, Directories, and Filenames
- Walking Directory Trees
- Grabbing Data from the Web
- Sending e-mail
- Math Functions
- Random Values
- Dates and Times
- Zipped Archives
- Introduction to Python Classes
- About o-o Programming
- Defining Classes
- Constructors
- Instance Methods
- Properties
- Class Methods and Data
- Static Methods
- Private Methods
- Inheritance
- Untangling the nomenclature
Prerequisites
Before Taking this Class
Working/user level knowledge of an operating system such as Linux, Windows, or MacOS. Basic skill with at least one other programming language is desirable.Setup Requirements
Software/Setup For this Class
Python 3 or higher
Onsite Training
Do you have five (5) or more people needing this class and want us to deliver it at your location?