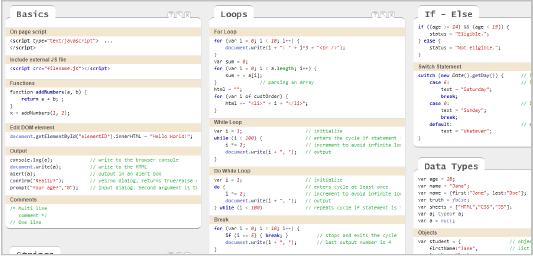
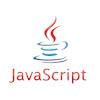
JavaScript Fundamentals Training
3 days (10:00 AM - 5:00 PM Eastern)
$1,295.00
Register for a live online class.
Details
Subjects Covered
Prerequisites
Setup Requirements
Details
Course Details
JavaScript enables interactive web pages and is an essential part of web applications. It is the essential technology behind Single Page Applications (SPA) and Progressive Web Applications (PWA). Modern usages of JavaScript extend beyond the browser to include creating web servers and other utility applications facilitated by programs such as NodeJS.
Students will learn to use JavaScript effectively to make their web applications more dynamic and functional and to reduce the number of roundtrips to the server. Students will learn JavaScript syntax, how to work with variables, flow control logic, and AJAX calls, as well as gain an advanced understanding of how JavaScript objects and functions work. They will learn the latest JavaScript features added with ES2015, as well as how to make use of next-generation language features that are not yet supported by major browsers.
Subjects Covered
- Introduction
- Modern Application Architecture
- Language Versions and Browser Support
- JavaScript Overview
- JavaScript Syntax
- Variables and Types
- Strings and Template Strings
- Operators
- Objects
- Arrays
- Conditionals and Loops
- Truthy and Falsey
- Functions
- Scope, var and let
- Handling Exceptions
- JavaScript in the Browser
- The script Tag
- Scripting Best Practices
- The Window Object
- Debugging JavaScript
- The DOM
- What is DOM?
- Node and NodeList
- Searching the DOM
- Navigating the DOM
- Working with Attributes
- NodeList vs. Array
- Modifying the DOM
- CSS Selectors
- Event Handling
- DOM Events in the Browser
- Connecting Event Handlers
- Event Parameters
- load vs DOMContentLoaded
- Event Propagation
- Event Delegation
- Objects in Depth
- Object literals
- Property and Method notation
- Adding and Removing Members
- Iterating over Properties
- Execution Context and “this”
- Object Destructuring
- Spread Operator
- Namespaces
- Constructor Functions
- Prototypes and Inheritance
- Built-in JavaScript Objects
- Date
- RegExp
- Error
- String
- Number
- Array
- Math
- Arrays
- Array Literals
- Looping over Arrays
- Finding Elements
- Filtering and Mapping
- Reducing
- Spread Operator
- Functions
- Function Declaration vs. Function Expression
- Hoisting
- Default Parameters
- Arguments and Variadic Functions
- Call and Apply
- Arrow Functions
- Rest Operator
- Closures
- Modularization
- Data Hiding
- Privileged Methods
- IIFEs
- The Module Pattern
- ES6 Modules
- 3rd Party Module Loaders
- AJAX
- AJAX Examples
- The XHR Object
- Handling Responses and Content
- Promises
- AJAX Limitations
- Client-side Data Storage
- localStorage
- sessionStorage
- IndexedDB
- Next-Generation Features
- Transpiling with Babel
- Class Syntax
- Property Descriptors
- Freezing and Sealing Objects
- Symbols
Prerequisites
Before Taking this Class
Experience in HTML is required and experience in CSS and programming would be beneficial.Setup Requirements
Software/Setup For this Class
Students will need a text editor (we recommend Visual Studio Code, but any will do) and NodeJS installed.
Onsite Training
Do you have five (5) or more people needing this class and want us to deliver it at your location?